JSCodeshift and ASTs
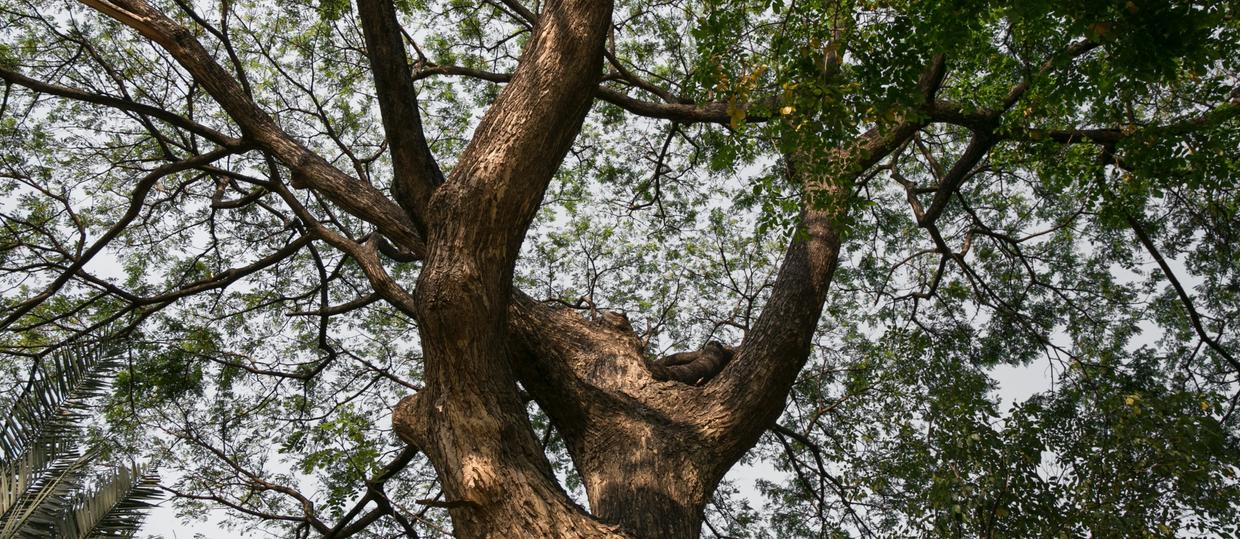
I have been working on some open-source tools for CanJS. I am working on the migration tool which is used to help upgrade projects to the latest version. We use jscodeshift to handle these migrations and this was my first time using them and writing them.
jscodeshift allows you to create complex transformations that can read the source file and parse that into an Abstract Syntax Tree (AST). We can then manipulate it and regenerate the source code.
AST Explorer
AST Explorer has been an extremely useful tool in helping me understand AST's and I've been using it to create my transforms live in the browser. It was very helpful to me to see the transformations happening as I was writing the transformation.
To enable the transforms within AST Explorer toggle the transform option within the top navigation bar. By turning this on you can select different types of transformations, as I am working with jscodeshift I use that option.
Examples
Let's walk through a simple transformation that will transform CanJS Component into a StacheElement class.
1Component.extend({2 tag: 'my-app',3 view: `<h1>Hello World!</h1>`4})
Will become
1class MyApp extends StacheElement {2 static get view() {3 return `<h1>Hello World!</h1>`4 }5}6customElements.define('my-app', MyApp)
Here is a gist with the code used to do the transformation in its most basic form:
Let's try to break this down a little, passing jscodeshift the source file will generate the AST and we then call the .find method which allows us to traverse and find something specific within the tree. It will return a Collection which you can iterate through and modify.
1.find(j.CallExpression, {2 callee: {3 type: 'MemberExpression',4 object: {5 name: 'Component'6 },7 property: {8 name: 'extend'9 }10 }11})
In the above code, we are looking for a CallExpression which has a specific callee and we can specify the type and names of the callee, in this instance, we are looking for Component.extend.
Once we have the results we can iterate over them and modify to our heart's content 😊.
We know the type of result we are going to get and the shape of it, so we can iterate over the properties of the object enabling us to add these back after we have replaced the CallExpression with a Class declaration.
We want to restore the view property and will add this to the class as a static getter property. Here we keep a reference to the view's path.
1path.value.arguments[0].properties.forEach(p => {2 if (p.key.name === 'view') {3 viewProp = p4 }5})
Now we can replace the CallExpression with a Class declaration:
1j(path).2replaceWith(j.classDeclaration(3 j.identifier(className),4 j.classBody([5 j.methodDefinition('get', j.identifier('view'),6 j.functionExpression(null, [], j.blockStatement([j.returnStatement(viewProp.value)])), true)7 ]), j.identifier('StacheElement')))
The first identifier is the name of the class, the second identifier is optional and it's for adding the name of the superClass. For the classBody we will just add what was previously the value of the view property on the CallExpression.
Full working example can be seen here.
Notes
When you wish to create a Node, use the camelCase name, and when you wish to look up a Node use the PascalCase name. For example, if you wish to find a classDeclaration you would do:
1j(file.source) .find(j.ClassDeclaration)
But if you wanted to create a class you would do:
1const classDeclaration = j.classDeclaration(...)
Voilà we have a simple transform!!
Thanks for following along and thanks for reading.