ES6 Tips & Tricks
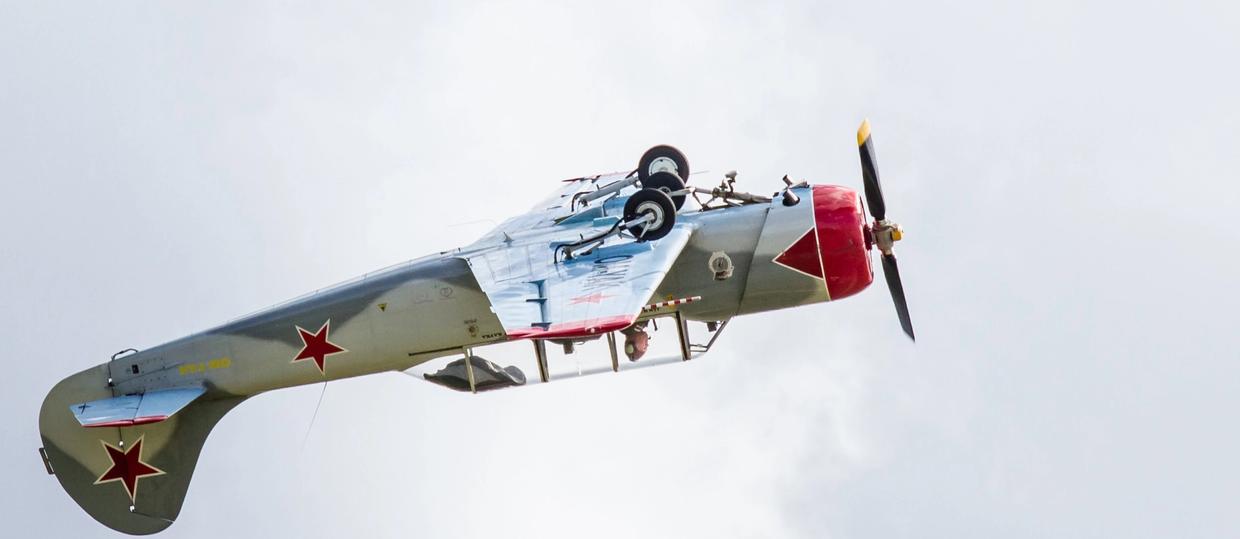
I wanted to put together a few useful tips & tricks that I find handy, mostly for me as a resource to look back at when I inevitably forget. I am not going to write about all the ES6 features as most of them are pretty well known, this is more of a handy resource for a few things I find useful.
Optional Object Properties
This is one I use fairly often as I find it a neat way to add conditionally properties to an object without lots of if statements.
1const hobbies = ['hiking']2const address = null3const hasHobbies = hobbies.length > 04const person = {5 name: 'Matt',6 age: 30,7 // Add this if there are hobbies8 ...(hasHobbies && { hobbies }),9 // Add address if exists, otherwise use a default10 ...(address ? { address } : { address: defaultAddress })11}
I think having all the potential properties around an object at the place of the definition of the object makes it easier at a glance to know what that object contains. Whereas the following I think is less glanceable:
1const hobbies = ['hiking']2const address = null3const person = {4 name: 'Matt',5 age: 306}7if (hobbies.length > 0) {8 person.hobbies = hobbies9}10if (address) {11 person.address = address12} else {13 person.address = defaultAddress14}
Object Spread Property Removal
This is a nice way to discard a property from an object, create a helper function that you can pass an object and which property you wish to remove and it will return the result. This makes use of the Spread Syntax.
1const person = {2 fname: 'Matt',3 lname: 'Chaffe',4 age: 305}67function removePropertyFromObject(obj, propertyName) {8 const {9 [propertyName]: discard, ...remainingProperties10 } = obj11 return remainingProperties12}13removePropertyFromObject(person, 'age') //-> { fname: 'Matt', lname: 'Chaffe' }
Array Concatenation
A way to concatenate arrays making use of the Spread Syntax, to empty the array being spread into the new array being created.
1const array1 = [1, 2, 3]2const array2 = [4, 5, 6]3const result = [...array1, ...array2] //-> [1, 2, 3, 4, 5, 6]
Template Literals
This is pretty commonly used, but I wanted to share it regardless because I think it's a really concise way to concatenate strings.
1const firstName = 'Matt'2const lastName = 'Chaffe'3const fullName = `${firstName} ${lastName}` // Matt Chaffe
Over the more traditional way of string concatenation:
1const firstName = 'Matt' const lastName = 'Chaffe' const fullName = firstName + ' ' + lastName // Matt Chaffe const firstName = 'Matt'2const lastName = 'Chaffe'3const fullName = firstName + ' ' + lastName // Matt Chaffe
Thanks for reading!